Blog
How to Connect Servlet Program with MySQL Database
- March 6, 2022
- Posted by: jcodebook
- Category: Servlet Tutorials
In this blog, you will learn How to Connect Servlet Program with MySQL Database. In addition to that, you will also learn How to download and configure MySQL Java Connector into the Eclipse Web Project.
Prerequisites
Install the following software
- Eclipse IDE
- MySQL Database
Here are the steps to connect Servlet with MySQL Database.
Step-1: Create a Database Schema and a Data Table
Open the MySql database and write the following Queries to create a User data table in the skillplusplus database.
create database skillplusplus;
use skillplusplus;
create table User(Name varchar(500), Email varchar(500));
insert into User values('James', '[email protected]');
insert into User values('Amit', '[email protected]');
Step-2: Download MySQL Java Connector
Use the link https://downloads.mysql.com/archives/c-j/ to download the MySQL Java connector as shown below.
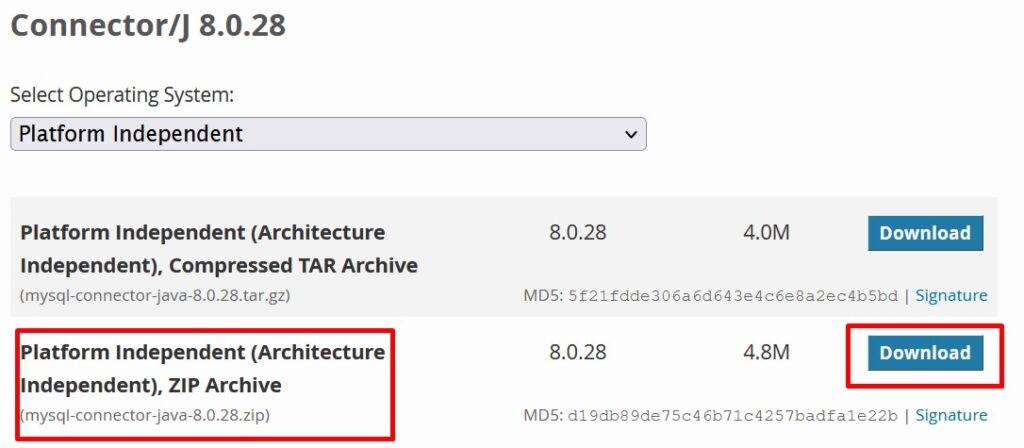
Extract the mysql-connector-java-8.0.28.zip file and copy the mysql-connector-java-8.0.28.jar and paste it into the following project folder as shown in the Figure below.
For example, your web project name is ‘SkillPlusPlus’.
SkillPlusPlus ->WebContent ->WEB-INF ->lib
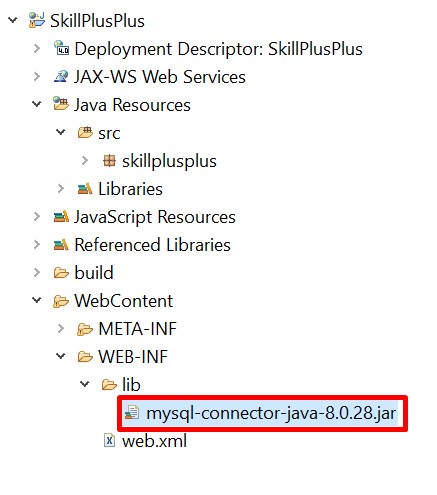
After this, add mysql-connector in the project build path as shown in the figure below.

Now, All Set.
Step-3: Create a Servlet Class
Create a MySQLDemo.java file as shown below
package skillplusplus;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/MySQLDemo")
public class MySQLDemo extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter pw=response.getWriter();
//initializing connections
Connection con=null;
Statement stmt=null;
ResultSet rs=null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
con = DriverManager.getConnection("jdbc:mysql://localhost:3306/skillplusplus","root","root");
stmt = con.createStatement();
rs = stmt.executeQuery("select * from User");
while (rs.next()) {
pw.print("<br>"+rs.getString("Name")+", "+rs.getString("Email"));
}
}// End of try block
catch(Exception e) {e.printStackTrace();}
}
}
Run the above java file and the following output will be displayed.
Output
