In this lesson, you will learn.
- Introduction
- File Stream Classes
- Example: Reading a File
- Example: Writing to a File
Introduction
To handle large volumes of data, we need some devices like hard disks to store data. A file stores the data in hard disks and contains read and write operations.
Generally, a C++ program involves two task
- Communication between the console unit and the program (Already discussed in the previous lesson using cin and cout objects).
- Data communication between the program and disk files
Transferring the data between the program and disk files can be achieved by file handling concepts in C++.
So, to handle files, the file stream classes are used that supply the data through the input stream and receive it from the program as an output stream.
File Stream Classes
- In C++, file streams are used for input and output operations on files.
- The standard file stream classes are part of <fstream> header.
- The main classes for file stream handling in C++ are
ifstream
,ofstream
, andfstream
.
The following Figure shows the file stream class hierarchy.
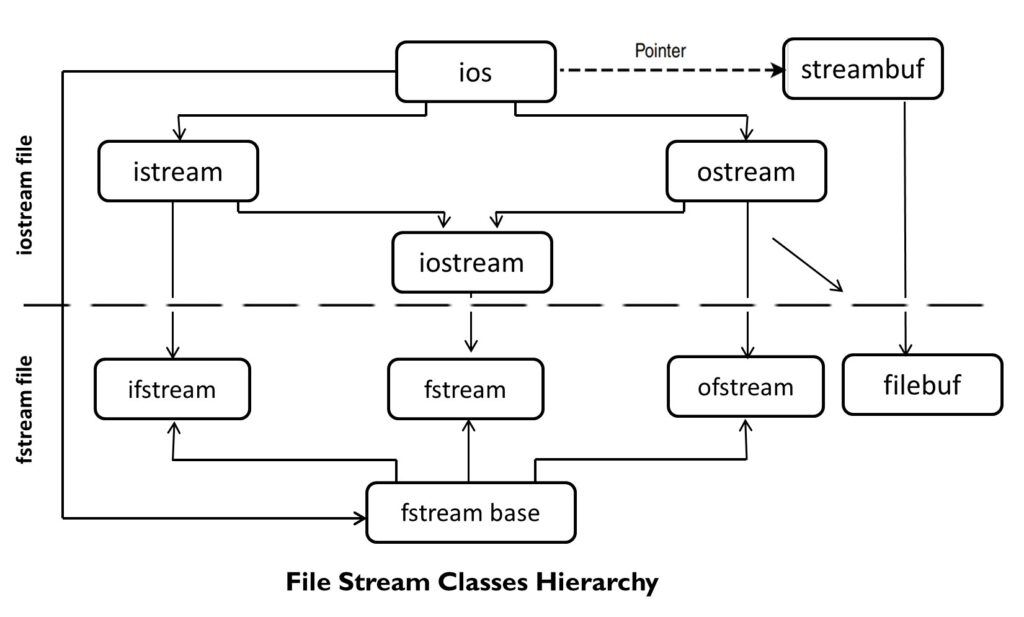
S.N. | Class | Description |
---|---|---|
1. | filebuf | Sets the file buffer to read and write. |
2. | fstreambase | It is a base class for ifstream, stream, and ofstream and contains open() and close() method. |
3. | ifstream(Input File Stream) | Used for reading data from files and contains open() method with default input mode. Inherits the get(), getline(), read() functions from istream. |
4. | ofstream(Output File Stream) | Used for writing data to files. Inherits put(), write() function from ostream. |
5. | fstream(File Stream) | Can be used for both reading and writing data to files |
Example 1: Reading a File
//FileStreamReadingEx1.cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream inputFile("example.txt"); // Open file for reading
if (inputFile.is_open()) {
// Read data from the file
string line;
while(getline(inputFile, line)) {
cout << line <<endl;
}
inputFile.close(); // Close the file
} else {
cerr << "Error opening file!" <<endl;
}
return 0;
}
Output
Hello, File I/O!
This is a new line.
Explanation
ifstream inputFile("example.txt");
This line creates an ifstream
object named inputFile
and opens the file named “example.txt” for reading.
If the file does not exist or cannot be opened for some reason, you can check the state of the stream using the is_open()
member function.
getline() function reads the file line by line and stores it in the line string.
inputFile.close();
Finally, after reading the file, it’s good practice to close the file using the close()
member function to free up resources associated with the file stream.
Example 2: Writing to a File
//FileStreamWritingEx1.cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outputFile("example.txt"); // Open file for writing
if (outputFile.is_open()) {
// Write data to the file
outputFile<< "Hello, File I/O!" <<endl;
outputFile<< "This is a new line.";
outputFile.close(); // Close the file
cout << "File written\n";
} else {
cerr << "Error opening file!" << std::endl;
}
return 0;
}
Output
File written
Note: Open the file “example.txt” and check whether the content is written or not.
Example 3: Writing Data
//FileStreamWritingEx2.cpp
#include <fstream> //for file I/O
#include <iostream>
#include <string>
using namespace std;
int main()
{
char ch = 'x';
int j = 77;
double d = 6.02;
string str1 = "File Handling"; //strings
string str2 = "Using C++";
ofstream outfile("fdata.txt"); //create ofstream object
outfile<< ch //insert (write) data
<< j
<< ' ' //needs space between numbers
<< d
<< str1
<< ' ' //needs spaces between strings
<< str2;
cout << "File written\n";
return 0;
}
Output
File written
Note: Open the file “fdata.txt” and check whether the content is written or not.
Explanation
ofstream outfile(“fdata.txt”);
An object called outfile to be a member of the ofstream class is created and opens the file “fdata.text” on the disk. If the file doesn’t exist, it is created. If it does exist, it is truncated and the new data replaces the old.
Example 4: Reading Data
//FileStreamReadingEx2.cpp
#include <fstream> //for file I/O
#include <iostream>
#include <string>
using namespace std;
int main()
{
char ch;
int j;
double d;
string str1;
string str2;
ifstream infile("fdata.txt"); //create ifstream object
//extract (read) data from it
infile >> ch >> j >> d >> str1 >> str2;
cout << ch << endl //display the data
<< j << endl
<< d << endl
<< str1 << endl
<< str2 << endl;
return 0;
}
Output
x
77
6.02
File
Handling
End of the lesson….enjoy learning
Student Ratings and Reviews
There are no reviews yet. Be the first one to write one.
Submit a Review
You must be logged in to submit a review.