Learn Java Programming
- Description
- Curriculum
- Reviews
Java is one of the world’s most important and widely used computer programming languages. Furthermore, it has held that distinction for many years. Unlike some other computer languages whose influence has waned with time, Java has grown stronger.
Java leaped to the forefront of Internet programming with its first release. Each subsequent version has solidified that position. Today, it is still the first and best choice for developing web-based applications.
This comprehensive Java Programming course is designed to equip learners with a solid foundation in Java, one of the most versatile and widely used programming languages. Whether you are a beginner with no prior coding experience or an experienced developer looking to enhance your skills, this course provides a step-by-step learning path to master Java programming.
-
1Overview of JavaPreview 5 Minutes
-
2Understanding the Features of JavaPreview 15 Minutes
-
3Difference between Java and C++Preview 10 Minutes
-
4Architecture of JavaPreview 20 Minutes
-
5Downloading and Installing JavaPreview 3:26
-
6Installing Eclipse IDEPreview Text lesson
-
7Creating Hello World Java ProgramPreview 15 Minutes
-
8Overview of Java and Its Features15 questionsThis quiz is based on the introduction to java and its features.
-
14Command Line Arguments in JavaPreview Text lesson
-
15Exercises: Java Command Line ArgumentsText lesson
-
16Taking Input using Scanner Class in JavaText lesson
-
17Taking Input Using BufferedReader and InputStream Reader in Java10 Minutes
-
18Bonus! Taking Input Using Console ClassText lesson
-
19Working With Inputs in Java11 questionsThis quiz assesses the knowledge of different input methods in Java.
-
20Overview of Java OperatorsPreview Text lesson
-
21Arithmetic Operators in JavaText lesson
-
22Relational Operators in JavaText lesson
-
23Logical Operators in JavaText lesson
-
24Bitwise Operators in JavaText lesson
-
25Shift Operators in JavaText lesson
-
26The Ternary (?) Operator in JavaText lesson
-
27Operator Precedence in JavaText lesson
-
28Introduction to Operators in Java3 questions
-
29Java Decision Making using If Else StatementsPreview Text lesson
-
30Switch Statements in JavaText lesson
-
31Working with do-while loop in JavaText lesson
-
32Working with While Loop in JavaText lesson
-
33Understanding foreach loop in JavaText lesson
-
34Working with Jump Statements in JavaText lesson
-
35Working with for loop in JavaText lesson
-
39Creating Class and Object in JavaPreview Text lesson
-
40Exploring Methods in JavaText lesson
-
41Understanding Static Keyword in JavaText lesson
-
42Method Overloading in JavaText lesson
-
43Understanding Constructors in JavaText lesson
-
44Parameterized Constructor In JavaText lesson
-
45Constructor Overloading in JavaText lesson
-
46Understanding Copy Constructor in JavaText lesson
-
47Understanding This Keyword in JavaText lesson
-
48Passing Objects as a Parameter in a Method in JavaPreview Text lesson
-
49Returning an Object from a Method in JavaText lesson
-
50Passing Object as a Parameter to a ConstructorText lesson
-
51Returning an Array of Object from a Method in JavaText lesson
-
52Returning 2D Array of Objects from a Method in JavaText lesson
-
53Implementing Encapsulation in JavaPreview Text lesson
-
54Understanding toString() Method in JavaText lesson
-
55Overview of Inheritance in JavaText lesson
-
56Working with Single Level Inheritance in JavaText lesson
-
57Working with Multilevel Inheritance in JavaText lesson
-
58Access Getters and Setter of Classes in Inheritance in JavaText lesson
-
59Parameterized Constructors and Super Keyword in JavaText lesson
-
60Understanding Method Overriding in JavaText lesson
-
61Understanding Dynamic Method Dispatch in JavaText lesson
-
62Using Final with InheritanceText lesson
-
63Understanding Hierarchical Inheritance in JavaText lesson
-
64Understanding Package in JavaPreview Text lesson
-
65AccessModifier in Inheritance in JavaText lesson
-
66Working with Abstract Classes in JavaText lesson
-
67Working with Interface in JavaText lesson
-
68Extending Interfaces in JavaText lesson
-
69Understanding Multiple Inheritance in JavaText lesson
-
70Understanding Diamond Problem of Multiple Inheritance in JavaText lesson
-
71Understanding Hybrid Inheritance in JavaText lesson
-
72Introduction to Strings in JavaPreview Text lesson
-
73Working with String Constructors in JavaText lesson
-
74Exploring Methods of String in Java: Part 1Text lesson
-
75Exploring Methods of String in Java: Part 2Text lesson
-
76Working with StringBuffer and StringBuilder Class in JavaText lesson
-
77Overview of Errors and Exceptions in JavaPreview Text lesson
-
78Handling Exception Using Try Catch Statements in JavaText lesson
-
79Multiple Catch Block and Nested Try-Catch Statements in JavaText lesson
-
80Working with Finally Block in JavaText lesson
-
81Exception Hierarchy, Checked and Unchecked Exception in JavaText lesson
-
82Understanding the Throw and Rethrowing in Exception in JavaText lesson
-
83Understanding the throws Keyword in JavaText lesson
-
84Creating Custom Exception Classes in JavaText lesson
-
85Understanding Assertions in JavaText lesson
-
86Applying Assertions to Inventory Management Project in JavaText lesson
-
87Understanding Additional Exception Featutres in JavaText lesson
-
88Overview of Stream Classes in JavaPreview Text lesson
-
89Creating File and Directory in JavaText lesson
-
90Writing to the File Using FileWriter and BufferedWriter Class in JavaText lesson
-
91Understanding Try with Resources Concept in JavaText lesson
-
92Reading From a File Using BufferedReader Class in JavaText lesson
-
93Reading a Text File Using Scanner ClassText lesson
-
94Deleting File and Text in JavaText lesson
-
95Reading and Writing on File using FileInputStream and FileOutputStream Class in JavaText lesson
-
96Understanding Serialization and Deserialization of Objects in JavaText lesson
-
97Overview of Multithreading Concept in JavaPreview Text lesson
-
98Introduction to Thread and Creating Main Thread in JavaText lesson
-
99Understanding The Life Cycle of a Thread in JavaText lesson
-
100Creating a Thread By Extending a Thread Class in JavaText lesson
-
101Understanding isAlive() and Join() Method of ThreadText lesson
-
102Creating Thread by Implementing Runnable Interface in JavaText lesson
-
103Understanding the Thread Priority in JavaText lesson
-
104Understanding The Thread Synchronization Concept in JavaText lesson
-
105Understanding Interthreaded Communication, Wait() and Notify() Method in JavaText lesson
-
106Understanding the Producer and Consumer Problem in JavaText lesson
-
116Introduction to AWT and Swing Components in JavaPreview Text lesson
-
117Creating a JFrame in Java SwingsText lesson
-
118Creating a JDialog in Java SwingsText lesson
-
119Creating a JPanel in Java SwingsText lesson
-
120Creating a JLabel in Java SwingsText lesson
-
121Creating JTextFields in Java SwingsText lesson
-
122Creating a JButton in Java SwingsText lesson
-
123Creating a JTable in JavaText lesson
-
124Creating a JTextArea in Java SwingsText lesson
-
125Creating a JCheckBox in Java SwingsText lesson
-
126Creating a JRadioButton in Java SwingsText lesson
-
127Creating a JList in Java SwingsText lesson
-
128Creating a JComboBox in Java SwingsText lesson
-
129Creating a JTabbedPane in Java SwingsText lesson
-
130Creating a JOptionPane in Java SwingsText lesson
-
131Creating a JMenuBar, JMenu , and JMenuItem in Java SwingsText lesson
-
132Overview of Event Handling Concept in JavaPreview Text lesson
-
133Implementing the Button Event using ActionListener in JavaText lesson
-
134Implementing the Keyboard Event using KeyListener in JavaText lesson
-
135Implementing the Mouse Event using MouseListener and MouseMotionListener in JavaText lesson
-
136Overview of JDBCPreview Text lesson
-
137Installing MySQL Database on Windows OSVideo lesson
-
138Introduction SQL QueriesText lesson
-
139Connecting Java Program With MySQL Database using JDBC APIText lesson
-
140How to Perform CRUD Operations on MySQL Database using JavaText lesson
-
141Displaying MySQL Data on Swing JTable in JavaText lesson
-
142Exploring Prepared Statement Interface and Its Methods in JavaVideo lesson
-
143Performing CRUD Operations on MySQL Database Using GUI Forms in JavaVideo lesson
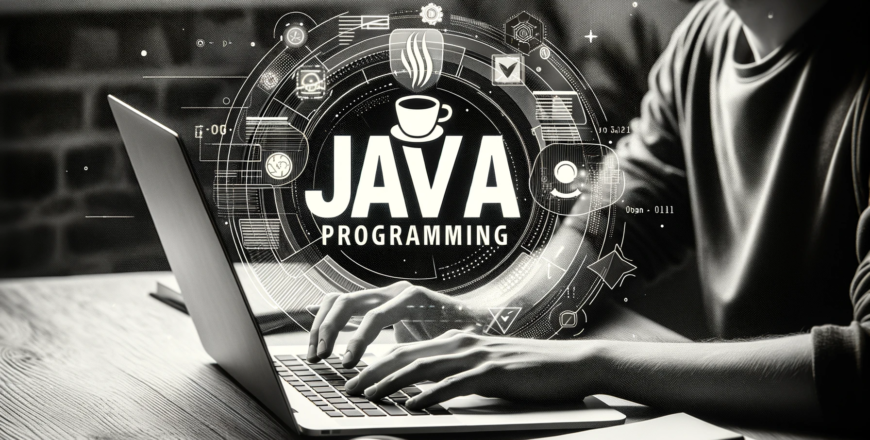